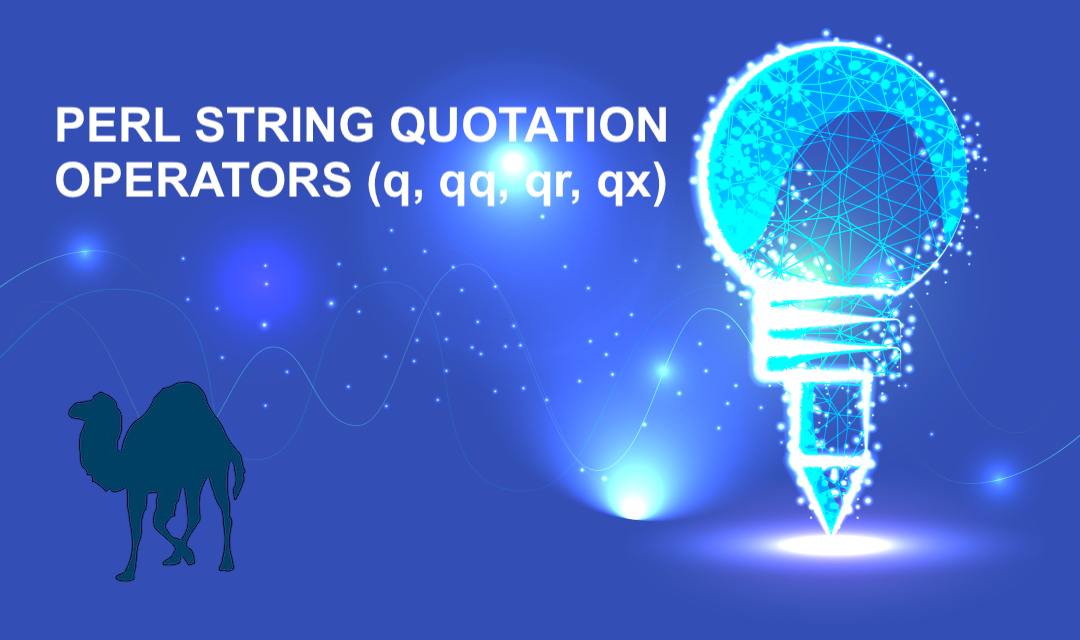
In scripting programming languages such as Perl, PHP, and Python use string quotation extensively. To include a single or double quote inside the string quotation, you'll have to escape the character with a backslash. Perl string quotation operators (q, qq and qx) let you avoid putting too many backslashes into quoted strings.
q/STRING/, or q(STRING) - Single Quotes, does not allow interpolation. qq/STRING/, or qq(STRING) - Double Quotes, allow interpolation. qr/EXPR/, or qr(EXPR) - Regexp-like quote qx/STRING/, or qx(STRING) - Backquote, executes external command inside backquotes.
The difference between a single quote and a double quote is an interpolation. A double quote allows the string to be replaced with the value of that variable inside the quote, while a single quote doesn't allow it.
The q operator (single quote) example:
#!/usr/bin/perl -w $name = "Cindy"; $str = q/This is $name's dog./; print $str;
The above script prints the following output.
This is $name's dog.
The qq operator (double quote) example:
#!/usr/bin/perl -w $name = "Cindy"; $str = qq(This is $name's dog.); print $str;
The above script prints the following output.
This is Cindy's dog.
The qr operator (regex quote) example:
#!/usr/bin/perl -w $regex = qr/[io]n/i; print $regex, "\n"; $string = "I am on chair in the room."; $string =~ s/$regex/XX/mg; print $string, "\n";
The above script prints the following output.
I am XX chair XX in the room.
Regular Expression Options:
m Treat string as multiple lines. s Treat string as a single line. (Make . match a newline) i Do case-insensitive pattern matching. x Use extended regular expressions. p When matching preserve a copy of the matched string so that ${^PREMATCH}, ${^MATCH}, ${^POSTMATCH} will be defined. o Compile the pattern only once.
The qx operator (backquote) example:
#!/usr/bin/perl -w $str = qx(date); print $str;
The above script prints the following output.
Wed May 18 19:30:36 CDT 2011
If the delimiter is an opening bracket or parenthesis, the final delimiter will be the corresponding closing bracket or parenthesis.
Conclusion
The q, qq, qr, and qx operators provide convenient ways to work with different types of strings (single-quoted, double-quoted), regular expressions, and external command execution in Perl.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment