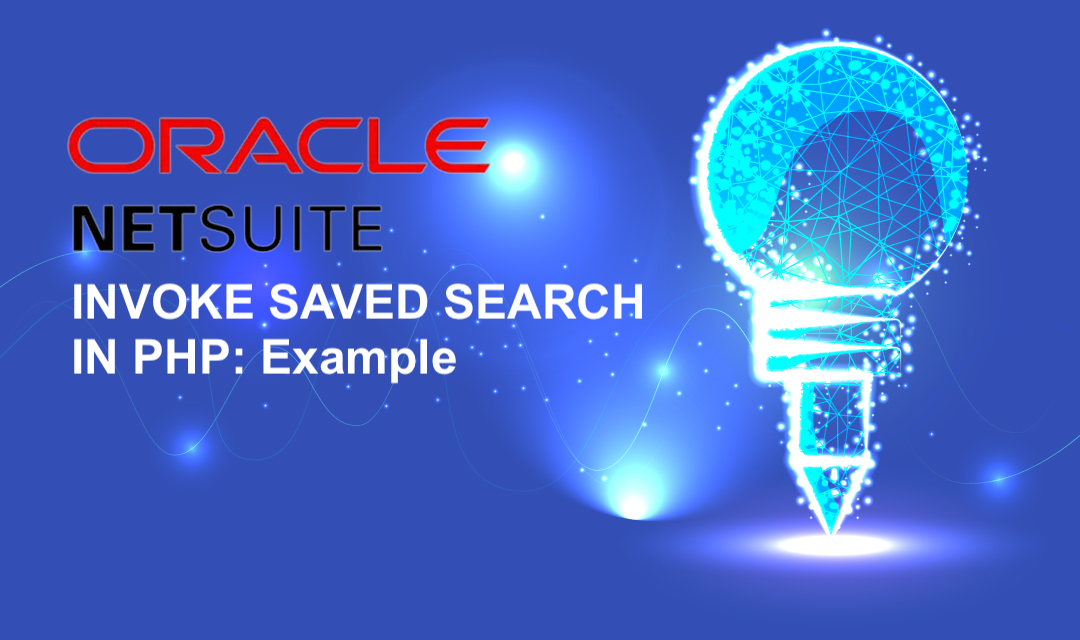
I've created a fair amount of PHP code to create custom searches on the Netsuite Platform. With the introduction of 2012.2 endpoints late last year, my examples have to be rewritten using the 2012.2 PHP Tool Kit API.
Here are a few examples written with v2011.2 API.
- Netsuite PHP Search Tutorials
- Netsuite PHPToolkit Web Service - Search ItemFulfillment Tutorial
- How to search custom fields in Netsuite with PHP
So, instead of rebuilding custom searches in PHP, I have decided to use Netsuite Saved Search this time. The caveat is that you must keep the Saved Search in your Netsuite System. If someone alters or deletes the Saved Search, your PHP script will no longer work. Other than that, it's perfectly OK to use Saved Search for your all search needs. Here are a couple of examples of searching through Inventory Items and Site Categories.
require_once ("NetSuiteService.php"); class Items { public function getSiteCategories() { $service = new NetSuiteService(); $service->setSearchPreferences(false, 1000, false); /** * Search Site Category and build a category list. */ $search = new SiteCategorySearchAdvanced(); $searchField = new SearchBooleanField(); $searchField->operator = "is"; $searchField->searchValue = "true"; $search->isInactive = $searchField; $request = new SearchRequest(); $request->searchRecord = $search; $searchResponse = $service->search($request); if (!$searchResponse->searchResult->status->isSuccess) { echo "SEARCH ERROR"; } else { echo "SEARCH SUCCESS, records found: " . $searchResponse->searchResult->totalRecords . "\n"; print_r($searchResponse->searchResult); } } public function get() { $service = new NetSuiteService(); $service->setSearchPreferences(false, 1000, false); $search = new ItemSearchAdvanced(); $search->savedSearchId = "693"; // Your SavedSearch ID. $request = new SearchRequest(); $request->searchRecord = $search; $searchResponse = $service->search($request); if (!$searchResponse->searchResult->status->isSuccess) { echo "SEARCH ERROR"; } else { echo "SEARCH SUCCESS, records found: " . $searchResponse->searchResult->totalRecords . "\n"; } } }
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment