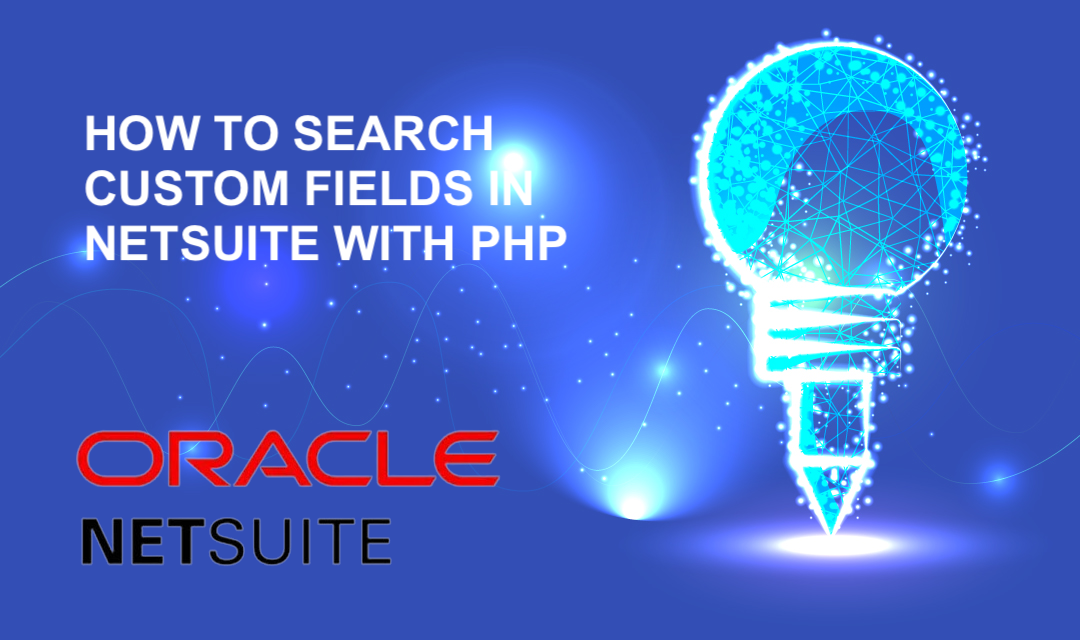
Netsuite allows an administrator to add custom fields and custom records via GUI. Custom fields are business-specific attributes defined by the company to tailor Netsuite. When a custom field is created in Netsuite, the administrator will assign an ID of the custom field and this ID becomes the internal ID of the custom field. To show internal ID on Netsuite GUI, it must be turned on by going to Home -> Set Preferences -> General -> Defaults, and checking the "Show Internal IDs" checkbox.
To illustrate with an example, let's assume that we defined a custom field called "custbody_admin" in the Purchase Order record type. Let's also assume that the value of "custbody_admin" is an employee-type record. Let's say we want to write a function that will take a PO number as an input, and return the email address of the "custbody_admin" (employee).
public function getAdminEmail($po) { global $myNSclient; $adminEmail = null; // Search for a purchase order from a PO number. $search = new nsComplexObject("TransactionSearchBasic"); $type = new nsComplexObject('SearchEnumMultiSelectField'); $type->setFields(array('searchValue' => array('_purchaseOrder'), 'operator' => 'anyOf')); $params = new nsComplexObject('SearchStringField'); $params->setFields(array('searchValue' => $poId, 'operator' => 'is')); $search->setFields(array ('type' => $type, 'tranId'=> $params)); $response = $myNSclient->search($search); if ($response->isSuccess) { $poObj = $response->recordList[0]; // Get the custom field list from the purchase order. $customFieldList = $poObj->getField('customFieldList'); $processor = null; // Loop through the custom field list, and search for the // custbody_admin (employee) record foreach ($customFieldList->getField('customField') as $customField) { if ("custbody_admin" == $customField->getField('internalId')) { $processor = $customField->getField('value'); $id = $processor->getField('internalId'); $recordRefs = array( new nsRecordRef(array("internalId" => $id, "type" => "employee"))); $recordList = $myNSclient->getList($recordRefs); $employee = $recordList[0]->record; $adminEmail = $employee->getField('email'); } } } return $adminEmail; }
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment