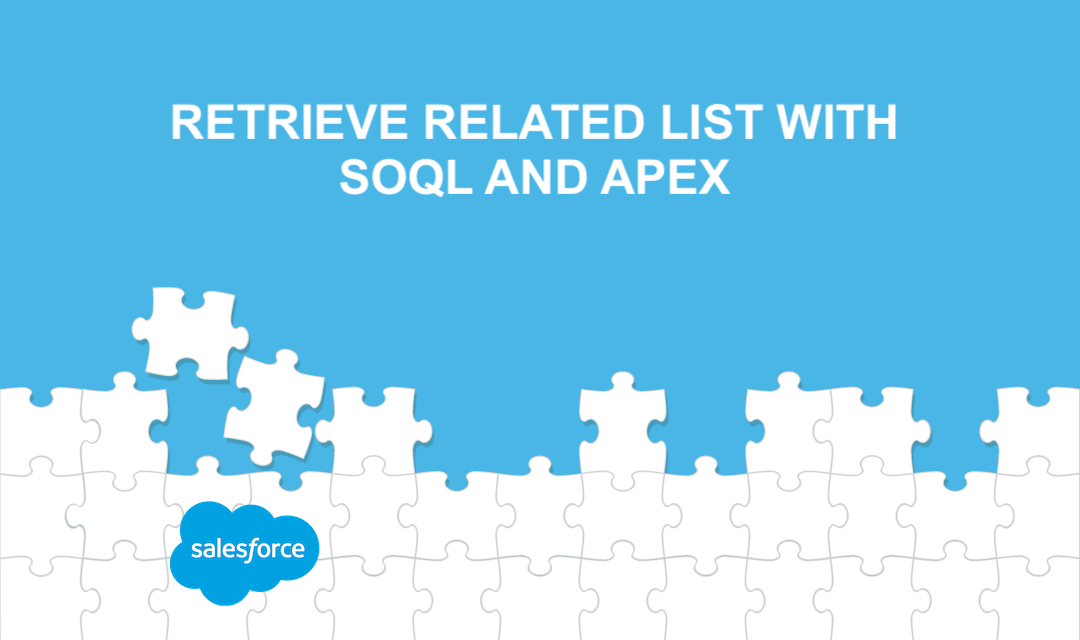
You are working with a Salesforce custom object, and you need to iterate through the related list. How do you retrieve the listed list with SOQL, and iterate through them with Apex? For illustration purposes, I'm going to use the Financial Force object, SCMC__Sales_Order__c. The SCMC__Sales_Order__c has a child relationship, SCMC__Purchase_Orders__r, as shown in the (Force.com IDE) screenshot below.
We have a custom field ("customerNote") in the Sales Order object, and we would like to copy this field into the same custom field ("customerNote") existing in the Purchase Order objects.
First we'll create an Apex Trigger on the Sales Order object:
trigger SalesOrder on SCMC__Sales_Order__c ( before insert, before update, after update) { if (Trigger.isBefore) { SalesOrderTriggerHandler.beforeInsertUpdate(Trigger.new); } else if (Trigger.isAfter) { SalesOrderTriggerHandler.afterUpdate(Trigger.new); } }
Now, that we created a Trigger we need to build business logic in an Apex Class -- SalesOrderTriggerHandler.
public with sharing class SalesOrderTriggerHandler { public static void beforeInsertUpdate(ListsalesOrders) { // some business logic for Before Insert, and Before Update trigger } public static void afterUpdate(List salesOrders) { for (SCMC__Sales_Order__c so : salesOrders) { // Retrieve the Customer Note field.. String customerNote = (String) so.get('customerNote__c'); // Only process if note field is not empty. if ((customerNote != null) && (customerNote.length() >0)) { // The SO object about to be written does NOT have the // reference to SCMC__Purchase_Orders__r, so we'll have // to Query again. for (SCMC__Sales_Order__c mySo : [Select id, (Select id, FTP_credentials__c from SCMC__Purchase_Orders__r) from SCMC__Sales_Order__c where Id = :so.Id]) { for (SCMC__Purchase_Order__c po : mySo.SCMC__Purchase_Orders__r) { po.customerNote__c = customerNote ; update po; } } } } } }
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment