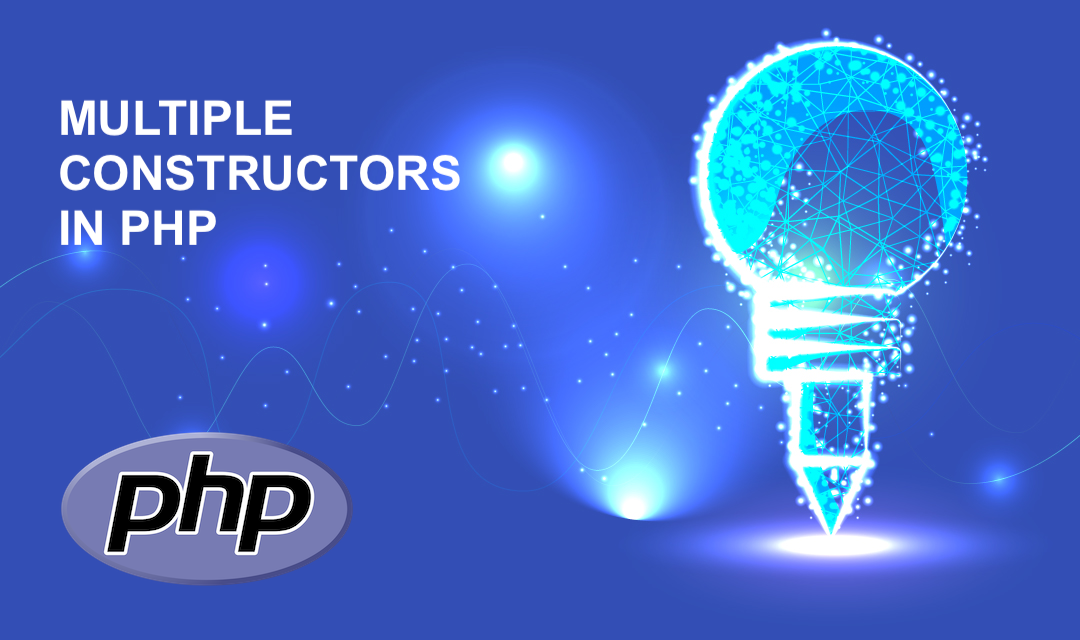
In most object-oriented programming languages such as Java, C++, and C#, you can define multiple constructors each having different signatures. The class calls the correct constructor based on the number of parameters, and data type of input parameters. In PHP5, however, you can have one and only one constructor. Constructor in PHP is defined with a reserved keyword, __construct(). If the __construct function doesn’t exist, PHP5 will search for the old-style constructor function by the name of the class.
Not being able to define multiple constructors in PHP5 is a big limitation. How do we work around this limitation, and create objects with varying initial values? There are a couple of ways to work around the problem, and here are the solutions.
1. Use func_get_args() and func_num_args()
class Example { function __construct() { $argv = func_get_args(); switch( func_num_args() ) { case 1: self::__construct1($argv[0]); break; case 2: self::__construct2( $argv[0], $argv[1] ); break; case 3: self::__construct2( $argv[0], $argv[1], $argv[2] ); } } function __construct1($arg1) { ... } function __construct2($arg1, $arg2) { ... } function __construct3($arg1, $arg2, $arg3) { ... } } $a = new Example("Argument 1"); $b = new Example("Argument 1", "Argument 2"); $b = new Example("Argument 1", "Argument 2", "Argument 3");
2. Use "static" Factory Pattern
The factory pattern allows for the instantiation of objects at runtime, which may be used to mimic the creation of each object with different factory methods. Instead of creating multiple constructors, you can define one factory method for each constructor.
class Example { public static function factory1($arg1) { } public static function factory2($arg1, $arg2) { } } $a = new Example::factory1("Argument 1"); $b = new Example::factory2("Argument 1", "Argument 2");
Conclusion
In PHP, a class can have only one constructor. However, you can achieve similar functionality by using the method above, or use the default values for constructor parameters such as parameter2=null, parameter3=null, and etc. This way, you can create instances of the class with different sets of parameters, effectively mimicking the behavior of multiple constructors.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment