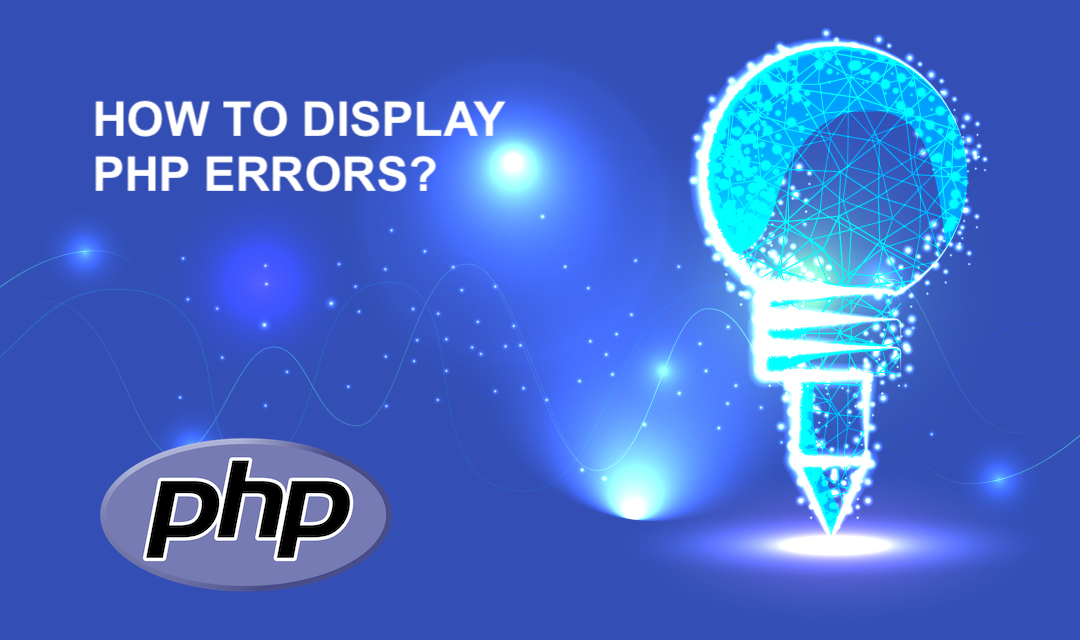
On a production server, you will definitely want to turn off errors from displaying on the screen due to security and usability reasons but what do you do when you're on a development machine trying to debug a malfunctioning PHP script? PHP gives a programmer the ability to control the logging of errors, and the types of errors to be displayed. You may enable PHP error display in one of two ways.
1. You may turn on the error display at the script level when a server-level error display is turned off.
ini_set('display_errors', 1); error_reporting(E_ALL);
** Although display_errors may be set at runtime (with ini_set('display_errors', 1)), it won't display anything if the script has fatal errors.
To display fatal errors, you'll need to register a shutdown function with the register_shutdown_function.
register_shutdown_function('shutdown'); function shutdown() { print_r(error_get_last()); }
2. You may turn on the error display at the server level by editing the php.ini file. The location of php.ini varies depending on the flavor of Linux you're running. On CentOS/RedHat, it is located in /etc folder, and on SuSE Linux, the php.ini is located in /etc/php5/apache2 folder.
display_errors = On error_reporting = E_ALL & ~E_NOTICE
3. You may suppress error at a line level with an @ operator. By having the @ operator on an expression, the error will be suppressed at the line level.
Here is the runtime configuration stored in the php.ini with the default settings.
; error_reporting is a bit-field. Or each number up to get the desired error ; reporting level ; E_ALL – All errors and warnings (doesn’t include E_STRICT) ; E_ERROR – fatal run-time errors ; E_WARNING – run-time warnings (non-fatal errors) ; E_PARSE – compile-time parse errors ; E_NOTICE – run-time notices (these are warnings which often result ; from a bug in your code, but it’s possible that it was ; intentional (e.g., using an uninitialized variable and ; relying on the fact it’s automatically initialized to an ; empty string) ; E_STRICT – run-time notices, enable to have PHP suggest changes ; to your code which will ensure the best interoperability ; and forward compatibility of your code ; E_CORE_ERROR – fatal errors that occur during PHP’s initial startup ; E_CORE_WARNING – warnings (non-fatal errors) that occur during PHP’s ; initial startup ; E_COMPILE_ERROR – fatal compile-time errors ; E_COMPILE_WARNING – compile-time warnings (non-fatal errors) ; E_USER_ERROR – user-generated error message ; E_USER_WARNING – user-generated warning message ; E_USER_NOTICE – user-generated notice message ; ; Examples: ; ; – Show all errors, except for notices and coding standards warnings ; error_reporting = E_ALL & ~E_NOTICE ; Print out errors (as a part of the output). For production websites, ; you’re strongly encouraged to turn this feature off and use error logging ; instead (see below). Keeping display_errors enabled on a production website ; may reveal security information to end users, such as file paths on your Web ; server, your database schema, or other information. display_errors = On
Note: PHP 5.3 or later, the default error_reporting value is E_ALL & ~E_NOTICE & ~E_STRICT & ~E_DEPRECATED. This setting does not show E_NOTICE, E_STRICT, and E_DEPRECATED level errors. Prior to PHP 5.3.0, the default value is E_ALL & ~E_NOTICE & ~E_STRICT. In PHP 4 the default value is E_ALL & ~E_NOTICE. To catch all errors during the development, "E_ALL" value is recommended.
NOTICE messages will warn you about possible bugs in your code. For example, the use of unassigned values is warned. It is extremely useful to find typos and to save time for debugging. NOTICE messages will warn you about bad style. For example, $arr[item] is better to be written as $arr['item'] since PHP tries to treat "item" as constant. If it is not a constant, PHP assumes it is a string index for the array.
In PHP 5, a new error level E_STRICT is introduced. Prior to PHP 5.4.0 E_STRICT was not included within E_ALL, so you would have to explicitly enable this kind of error level in PHP < 5.4.0. STRICT messages provide suggestions that can help ensure the best interoperability and forward compatibility of your code. These messages may include things such as calling non-static methods statically, defining properties in a compatible class definition while defined in a used trait, and prior to PHP 5.3 some deprecated features would issue E_STRICT errors such as assigning objects by reference upon instantiation.
Share this post
Leave a comment
All comments are moderated. Spammy and bot submitted comments are deleted. Please submit the comments that are helpful to others, and we'll approve your comments. A comment that includes outbound link will only be approved if the content is relevant to the topic, and has some value to our readers.
Comments (0)
No comment